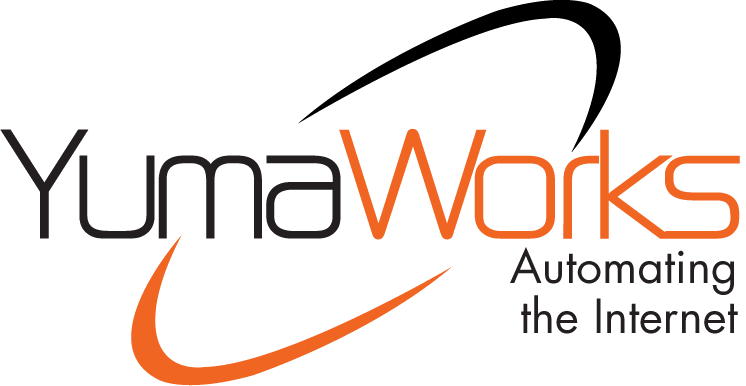
yp-show External Interface
The yangcli-pro client application supports an external vendor library that is loaded at boot-time by the yangcli_pro application. This library code is run in the context of the main yangcli-pro process. It is used to hook vendor-specific functions into the yangcli application,.
The following tasks are supported by the yp-yangcli-show library interface:
initialization
cleanup
hook in external yangcli model
hook in external yangcli interface
The default external show library is located in called
'libyp_show.so', it is located in the library path, such as
/usr/lib/yumapro/libyp_show.so
.
There is an example library in the 'libshow' directory of the YumaPro source tree. The 'example-show.cpp' file in the 'src' directory contains some stub code demonstrating how this library is used.
This file is installed as /usr/lib/yumapro/libexample-show.so
.
It must be copied or renamed in order to be used.
> sudo cp /usr/lib/yumapro/libexample-show.so /usr/lib/yumapro/libyp_show.so
If this worked correctly, then the 'show fan' command should be available when yangcli-pro or yp-shell is run:
> show fan
Report for Fan 1
put fan status here...
put more fan status here...
>
Mandatory YANG Module Definitions
For any added show commands, then there has to be a YANG module to define it.
This module must be loaded into the application using the
'ncxmod_load_module' function. The module example-fan.yang
is
ised in the libshow/src/example-show.cpp
file.
Mandatory yp-show API Functions
The following API functions should be defined in all yp-library code:
yp_show_init: Initialization function
yp_show_cleanup: Cleanup function
The following example shows the 'example-show.h' contents from the 'libshow/src' directory:
#ifndef _H_example_show
#define _H_example_show
/*
* Copyright (c) 2012 - 2022, YumaWorks, Inc., All Rights Reserved.
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
#ifndef _H_status_enum
#include "status_enum.h"
#endif
#ifdef __cplusplus
extern "C" {
#endif
/**
* @brief show init callback
*
* This callback is invoked twice; before and after CLI processing
*
* @return status: error will abort startup
*/
extern status_t yp_show_init (void);
/* show cleanup callback
* this callback is invoked once during agt_cleanup
*/
extern void yp_show_cleanup (void);
#ifdef __cplusplus
} /* end extern 'C' */
#endif
#endif
Terminal Customization APIs
The yp-show library can contain callbacks for altering the display output that is rendered to the standard output in interactive mode. This callback can alter the output before it is displayed to the user.
Command output is processed in the following order:
Raw command output using the specified display mode
yp-show terminal hook to alter output text
pipe command filtering
--More-- pagination
Register Callbacks
There are 2 callbacks that are registered, using one function from yangcli_term.h:
/********************************************************************
* FUNCTION ycli_register_term_callback
*
* Register a custom callback to process to server response
* or command output before it is processed by Pipe and More
*
* INPUTS:
* test_callback == test command callback
* exec_callback == exec command callback
*********************************************************************/
extern void
ycli_register_term_callback (term_test_cbfn_t test_callback,
term_cbfn_t exec_callback);
Test Function
The term_test_cbfn_t callback is invoked to determine if the command output should be processed by the “exec” callback.
/* external term handler test callback
* FUNCTION term_api_test_fn
*
* This API tests whether the term_api_fn is needed for this
* command or not;
*
* INPUTS:
* session_cb == session control block
* command_name == name of command being invoked or replied
* reply_output == TRUE if being called from reply handler
* FALSE if being called from local command output
* RETURNS:
* TRUE if command output API hook needed
* FALSE if ommand output API hook not needed
*********************************************************************/
typedef boolean
(*term_test_cbfn_t) (void *session_cb,
const char *command_name,
boolean reply_output);
Exec Function
The term_cbfn_t callback is invoked to alter the terminal output
/* external term handler callback
* FUNCTION term_api_fn
*
* This API alters the command or server output so
* yangcli_term can process the More and Pipe commands
*
* INPUTS:
* session_cb == session control block
* in_filespec == input filespec for API to process
* out_filespec == output file for API to write output
* RETURNS:
* status
*********************************************************************/
typedef status_t
(*term_cbfn_t) (void *session_cb,
const char *in_filespec,
const char *out_filespec);
yp-show Example
The following code can be found in the
libshow/src/example-show.cpp
source file.
Registration:
extern "C" status_t yp_show_init (void)
{
status_t res = NO_ERR;
log_debug("\nyp_show init\n");
ycli_register_term_callback(term_api_test_fn, term_api_fn);
return NO_ERR;
}
Example test callback:
/* example terminal output test API */
static boolean
term_api_test_fn (void *session_cb,
const char *command_name,
boolean reply_output)
{
/* current session control block if needed */
session_cb_t *cb = (session_cb_t *)session_cb;
(void)cb;
if (reply_output == FALSE) {
return FALSE;
}
if (!strcmp(command_name, "get") ||
!strcmp(command_name, "get-config")) {
return TRUE;
}
return FALSE;
} /* term_api_test_fn */
Example exec callback:
/* example terminal output API
* This API alters the command or server output so
* yangcli_term can process the More and Pipe commands
*/
static status_t
term_api_fn (void *session_cb,
const char *in_filespec,
const char *out_filespec)
{
/* current session control block if needed */
session_cb_t *cb = (session_cb_t *)session_cb;
(void)cb;
/* get file into a buffer
* this is just one way to process the input;
* a line-by-line approach could be used to save memory
*/
xmlChar *in_buff = NULL;
status_t res = ncx_file_to_buffer(in_filespec, &in_buff);
if (in_buff == NULL || res != NO_ERR) {
m__free(in_buff);
return res;
}
/* change all the '{' and '}' chars to spaces just
* as an example of altering the output
*/
xmlChar *p = in_buff;
while (*p) {
if (*p == '{' || *p == '}') {
*p = ' ';
}
p++;
}
/* write altered buffer out to a file */
res = ncx_buffer_to_file(out_filespec, in_buff);
m__free(in_buff);
return res;
} /* term_api_fn */